1. Injection
Injection vulnerabilities occur when untrusted data is sent to an interpreter as part of a command or query.
Example (SQL Injection):
SELECT * FROM users WHERE username = 'malicious' OR '1'='1';
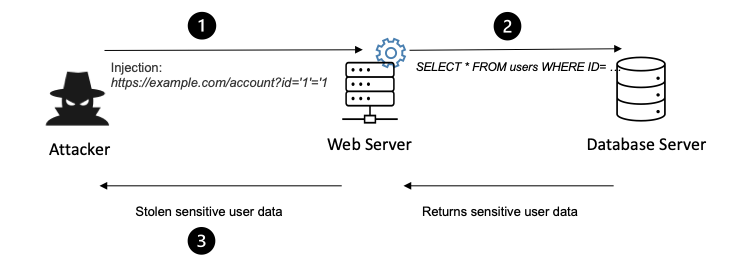
Injection attacks can lead to unauthorized access, data loss, or data corruption. To prevent injection vulnerabilities, use parameterized queries and input validation.
Reference: OWASP SQL Injection Prevention Cheat Sheet
2. Broken Authentication
Broken authentication occurs when authentication mechanisms are implemented incorrectly, allowing attackers to compromise user accounts.
Example:
if (userInputUsername === storedUsername && userInputPassword === storedPassword) {
// Allow access
} else {
// Deny access
}
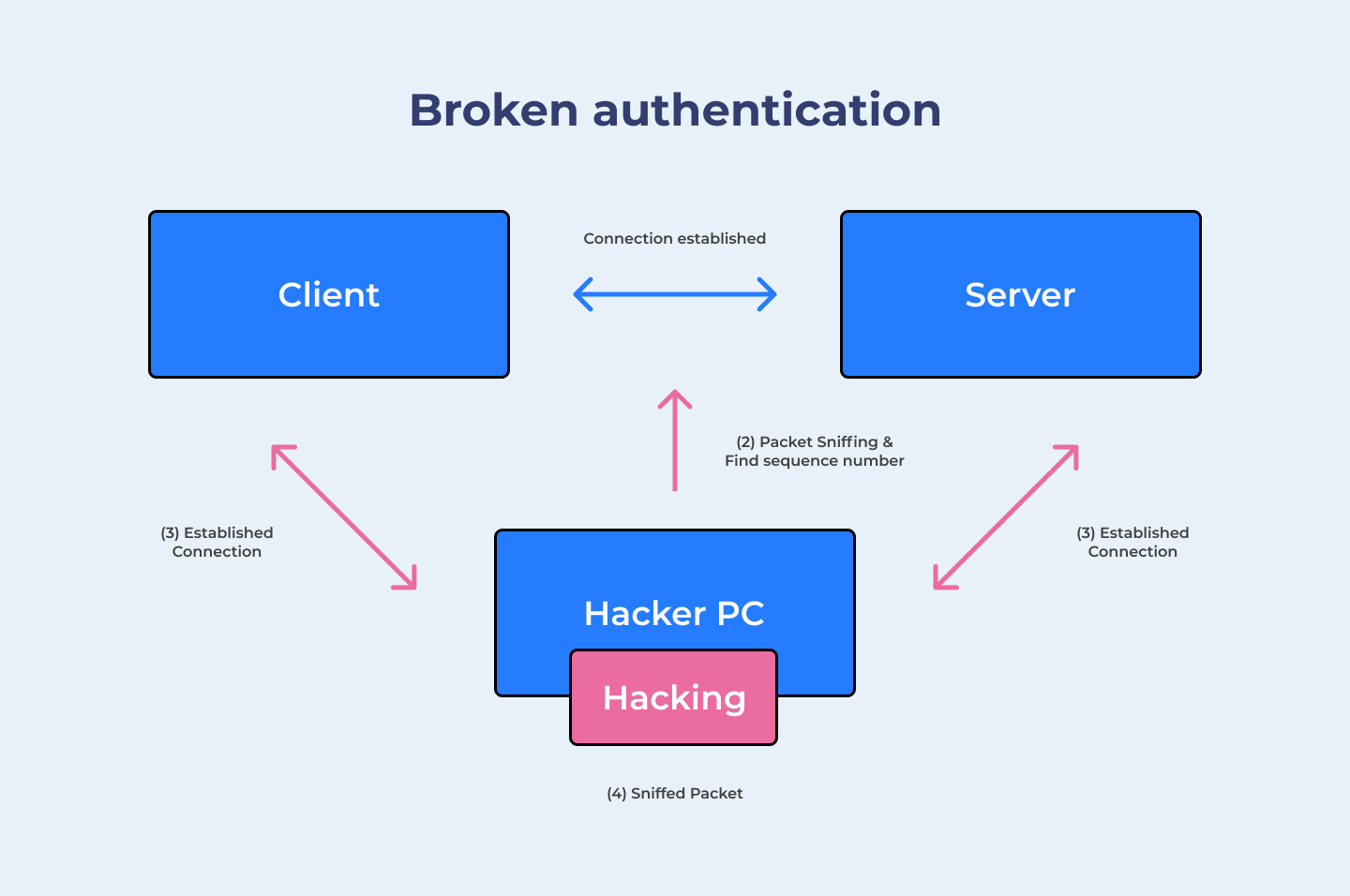
Ensure strong password policies, implement multi-factor authentication, and use secure session management to prevent broken authentication vulnerabilities.
Reference: OWASP Authentication Cheat Sheet
3. Sensitive Data Exposure
Sensitive data exposure occurs when sensitive information is not adequately protected, leading to unauthorized access.
Example:
// Storing passwords in plain text
const hashedPassword = hash(userInputPassword);
saveToDatabase(userInputUsername, hashedPassword);
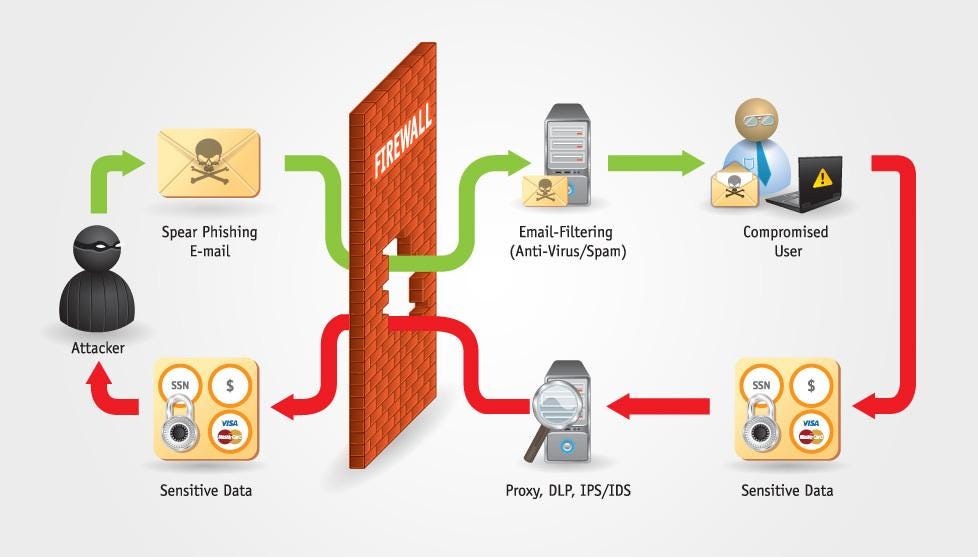
Encrypt sensitive data, use secure communication channels, and avoid storing unnecessary information to prevent sensitive data exposure.
Reference: OWASP Sensitive Data Exposure
4. XML External Entities (XXE)
XXE occurs when an application parses XML input containing a reference to an external entity, leading to disclosure of internal files, denial of service, or remote code execution.
Example:
]>
&xxe;

Prevent XXE by disabling external entity references, using a safe XML parser, and validating XML input.
Reference: OWASP XXE Prevention Cheat Sheet
5. Security Misconfigurations
Security misconfigurations occur when security settings are not properly implemented, allowing attackers to exploit vulnerabilities.
Example:
// Default admin credentials
username: admin
password: admin123
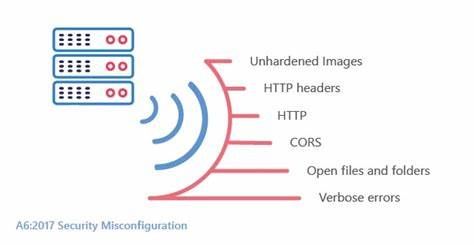
Regularly audit and review security configurations, follow the principle of least privilege, and apply secure default settings to prevent security misconfigurations.
Reference: OWASP Security Misconfigurations
6. Cross-Site Scripting (XSS)
XSS occurs when an application includes untrusted data on a web page, enabling attackers to execute malicious scripts in the context of a user's browser.
Example:
// Reflective XSS
http://example.com/search?query=<script>malicious_code</script>
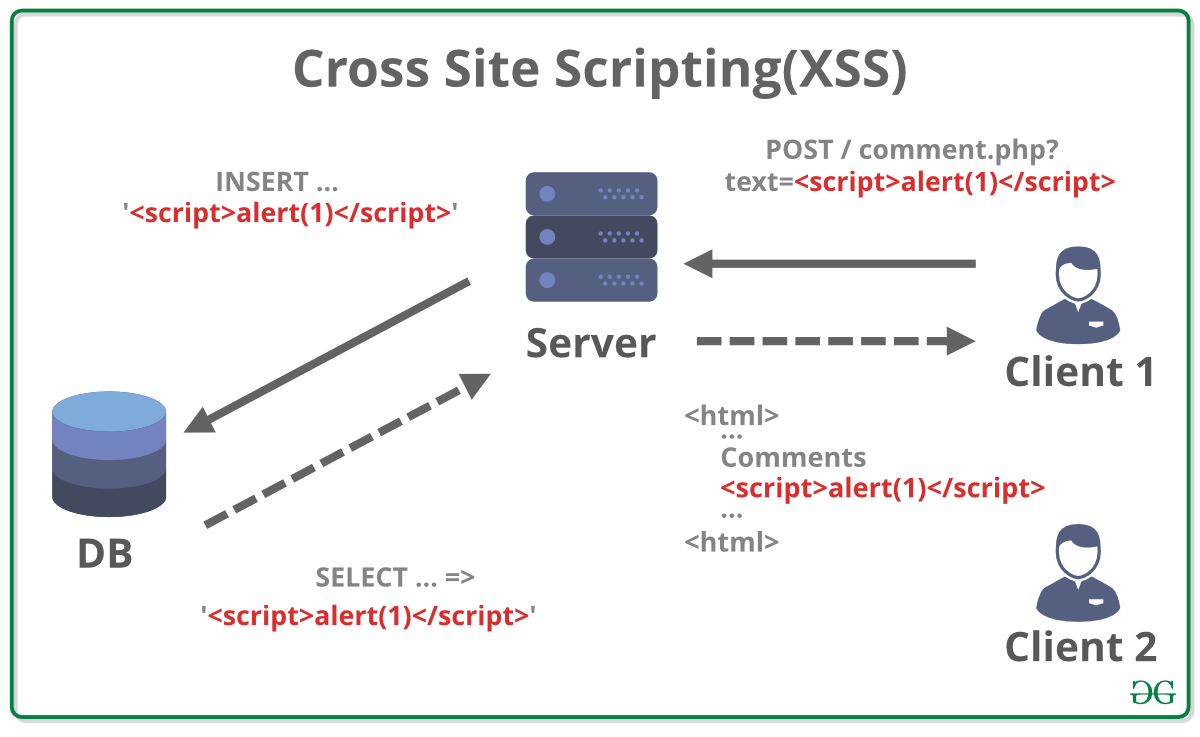
Prevent XSS by validating and sanitizing user input, using secure coding practices, and implementing Content Security Policy (CSP).
Reference: OWASP XSS Prevention Cheat Sheet
7. Insecure Deserialization
Insecure deserialization occurs when untrusted data is used to abuse the logic of an application, leading to remote code execution, privilege escalation, or DoS attacks.
Example:
// Deserialize user-provided data
const userProvidedData = unserialize(request.getParameter("data"));
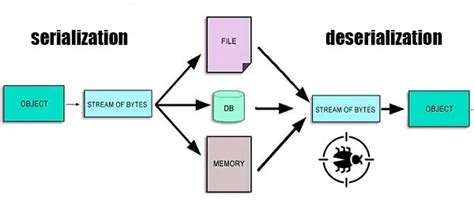
Prevent insecure deserialization by avoiding or properly validating serialized data, implementing integrity checks, and using secure deserialization libraries.
Reference: OWASP Deserialization Cheat Sheet
8. Components with Known Vulnerabilities
Using components with known vulnerabilities can expose applications to security risks. These vulnerabilities may include outdated libraries, frameworks, or third-party software.
Example:
// Using an outdated library
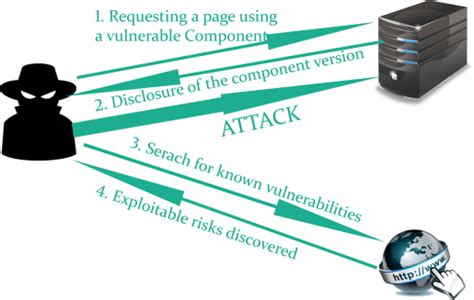
Regularly update and patch all components, monitor for security advisories, and use tools to identify and remediate known vulnerabilities.
Reference: OWASP Components with Known Vulnerabilities
9. Insufficient Logging & Monitoring
Insufficient logging and monitoring can prevent timely detection and response to security incidents, allowing attackers to go undetected.
Example:
// Inadequate logging
log.info("User authentication successful");
// No monitoring for suspicious activities

Implement robust logging, regularly review logs, and establish monitoring for suspicious activities to enhance incident response capabilities.
Reference: OWASP Logging Cheat Sheet
10. Insecure Direct Object References (IDOR)
IDOR occurs when an application provides direct access to objects based on user-supplied input, enabling unauthorized access to sensitive data.
Example:
// Insecure direct reference to user profile
http://example.com/view-profile?user=attacker
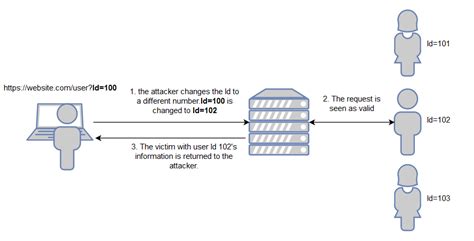
Prevent IDOR by enforcing proper access controls, validating user permissions, and using indirect object references rather than direct references.
Reference: OWASP Insecure Direct Object References
11. Unprotected APIs
Unprotected APIs can expose sensitive data and functionalities, leading to unauthorized access and potential exploitation.
Example:
// Exposing sensitive information via an unprotected API endpoint
GET /api/user/123
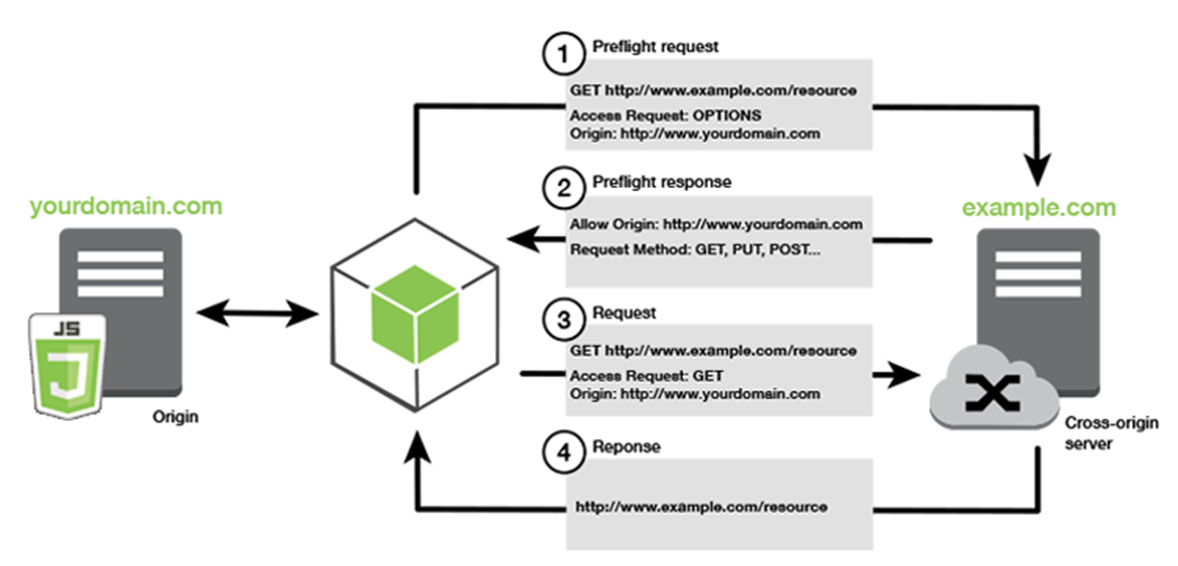
Secure APIs by implementing proper authentication mechanisms, authorization controls, and encrypting data in transit.
Reference: OWASP API Security Cheat Sheet
12. Insecure JWTs (JSON Web Tokens)
Insecure JWT implementation can lead to vulnerabilities such as unauthorized access, information leakage, and tampering of data.
Example:
// Insecure JWT with no signature verification
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c

Secure JWTs by using strong algorithms, validating tokens properly, and considering token expiration and revocation mechanisms.
Reference: OWASP JWT Cheat Sheet